- Advertorial & DMCA Protected -
Act immediately! Niki Profit is supercharging participants' earnings! Claim your spot before the
exclusive invite vanishes! 05:01
Grid Trading with Python: A Simple and Profitable Algorithmic Strategy
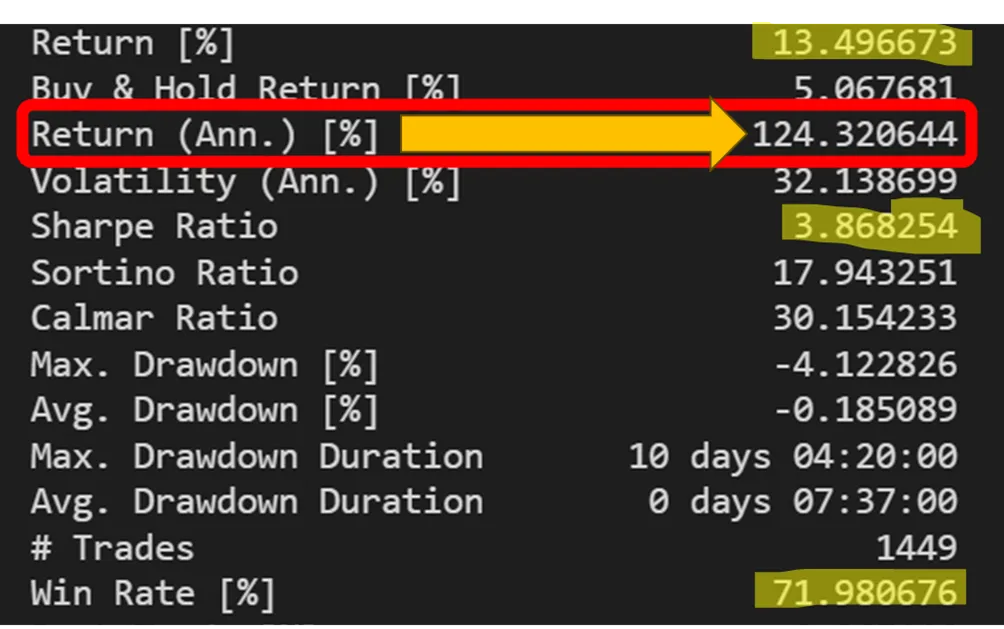
Grid trading is a sophisticated yet straightforward algorithmic trading strategy that exploits market volatility.
It involves placing buy and sell orders at predefined intervals above and below a set price line. This method capitalizes on small price fluctuations within markets, making it an ideal strategy during periods of relative price stability or mild trends.
Backtesting Grid Trading Strategies in Python
Backtesting is a critical step in validating any trading strategy, and Python provides a robust environment for this task. By simulating trading strategies on historical data, traders can gauge the effectiveness of their grid trading system before applying it to live markets.
Step 1: Setting Up the Environment
To get started, it is essential to have a well-configured Python environment. This includes installing necessary libraries such as `pandas` for data manipulation, `numpy` for numerical operations, and `matplotlib` for plotting, along with any trading-specific packages like `backtrader`.
import yfinance as yf
import pandas as pd
import numpy as np
import pandas_ta as ta
# Download the EUR/USD data for the last 59 days with a 5-minute interval
dataF = yf.download(“EURUSD=X”, start=pd.Timestamp.today() – pd.DateOffset(days=59), end=pd.Timestamp.today(), interval=‘5m’)
Step 2: Creating the Grid
The grid is the core of the strategy. It revolves around determining the price levels at which buy and sell orders will be placed. The grid structure requires careful planning to align with the market’s historical price movement and volatility levels. Once established, these grid levels dictate the automatic execution of trades.
grid_distance = 0.005
midprice = 1.065
def generate_grid(midprice, grid_distance, grid_range):
return np.arange(midprice – grid_range, midprice + grid_range, grid_distance)
grid = generate_grid(midprice=midprice, grid_distance=grid_distance, grid_range=0.1)
Defining Parameters:
grid_distance = 0.005
: This defines the distance between each grid line. It represents the price increment at which we will open new positions.midprice = 1.065
: This is the central price around which the grid is created. It can be set to the current market price or any other reference price.
Step 3: Generating Trading Signals
Trading signals derived from the grid structure trigger buying or selling decisions. As prices move and reach specific grid levels, corresponding trade signals prompt the execution of transactions. These signals help maintain the strategic flow of trades continuous during the trading period.
signal = [0] * len(dataF)
i = 0
for index, row in dataF.iterrows():
for p in grid:
if min(row.Low, row.High) < p and max(row.Low, row.High) > p:
signal[i] = 1
i += 1
dataF[“signal”] = signal
dataF[dataF[“signal”] == 1]
Step 4: Preparing for Backtesting
Preparing for backtesting involves gathering historical market data that accurately reflects past price movements. Format the data to align with your trading framework, ensuring the grid trading code can access it seamlessly. Proper preparation is essential for valid backtesting results.
dfpl = dataF[:].copy()
def SIGNAL():
return dfpl.signal
dfpl[‘ATR’] = ta.atr(high=dfpl.High, low=dfpl.Low, close=dfpl.Close, length=16)
dfpl.dropna(inplace=True)
Step 5: Implementing and Running the Backtest
With the environment ready and the data in place, the next step is implementing the backtest. This involves coding the grid strategy into your chosen backtesting platform, setting initial conditions, and running simulations to replicate practical trading scenarios.
from backtesting import Strategy
from backtesting import Backtest
import backtesting
class MyStrat(Strategy):
mysize = 50
def init(self):
super().init()
self.signal1 = self.I(SIGNAL)
def next(self):
super().next()
slatr = 1.5 * grid_distance # Stop loss distance
TPSLRatio = 0.5 # Take profit to stop loss ratio
if self.signal1 == 1 and len(self.trades) <= 10000:
# Sell position
sl1 = self.data.Close[-1] + slatr
tp1 = self.data.Close[-1] – slatr * TPSLRatio
self.sell(sl=sl1, tp=tp1, size=self.mysize)
# Buy position
sl1 = self.data.Close[-1] – slatr
tp1 = self.data.Close[-1] + slatr * TPSLRatio
self.buy(sl=sl1, tp=tp1, size=self.mysize)
# Running the backtest
bt = Backtest(dfpl, MyStrat, cash=50, margin=1/100, hedging=True, exclusive_orders=False)
stat = bt.run()
stat
Step 6: Analyzing the Backtest Results
Once complete, analyzing the backtest results offers insights into the strategy’s performance. Metrics such as profitability, trade frequency, and consistency over different time frames become apparent, informing potential adjustments and optimizations.
Summary:
Grid trading is particularly effective in range-bound markets, where conventional trend-following strategies may struggle. This comprehensive guide provides an approach to build and validate a basic grid trading model using Python.
Performance Metrics:
Performance metrics include net profitability, return on investment, and annualized returns. These indicators evaluate the strategy’s success in generating income over the test period.
-
- Final Equity: $136.02, starting from $50.
-
- Equity Peak: $156.59, showing potential for higher gains.
-
- Return: 172.04%, reflecting substantial profitability.
-
- Buy & Hold Return: 4.23%, demonstrating the strategy’s superior performance.
-
- Annualized Return: 37364.62%, extremely high due to the short backtest duration.
-
- Annualized Volatility: 46309.60%, indicating high risk and frequent trading.
Risk-Adjusted Metrics:
Risk-adjusted metrics, such as the Sharpe ratio and Sortino ratio, help assess how well the strategy compensates for the risk taken, highlighting its efficiency in managing trades’ inherent risks.
-
- Sharpe Ratio: 0.81, suggesting moderate risk-adjusted returns.
-
- Sortino Ratio: 1089.28, indicating excellent downside risk management.
-
- Calmar Ratio: 2194.58, reflecting high returns relative to maximum drawdown.
Drawdown:
Drawdown metrics reveal the peak-to-trough decline over a specified time, offering insights into the strategy’s potential risk and the investor’s capacity to withstand prolonged downturns.
-
- Max Drawdown: -17.03%, a significant but manageable decline.
-
- Avg Drawdown: -1.16%, relatively low.
-
- Max Drawdown Duration: Nearly 10 days, showing the longest recovery period.
-
- Avg Drawdown Duration: Less than 7 hours, indicating quick recoveries.
Trading Activity:
An active grid strategy will show high trading activity, entering and exiting positions frequently, capturing minor price swings. Analysis of trade counts and execution speed provides insights into operational efficiency.
-
- Number of Trades: 1698, high frequency due to the grid trading strategy.
-
- Win Rate: 73.03%, a very high success rate.
-
- Best Trade: 0.87%, consistent small gains.
-
- Worst Trade: -0.85%, controlled losses.
-
- Avg Trade: 0.10%, reflecting the cumulative effect of many small trades.